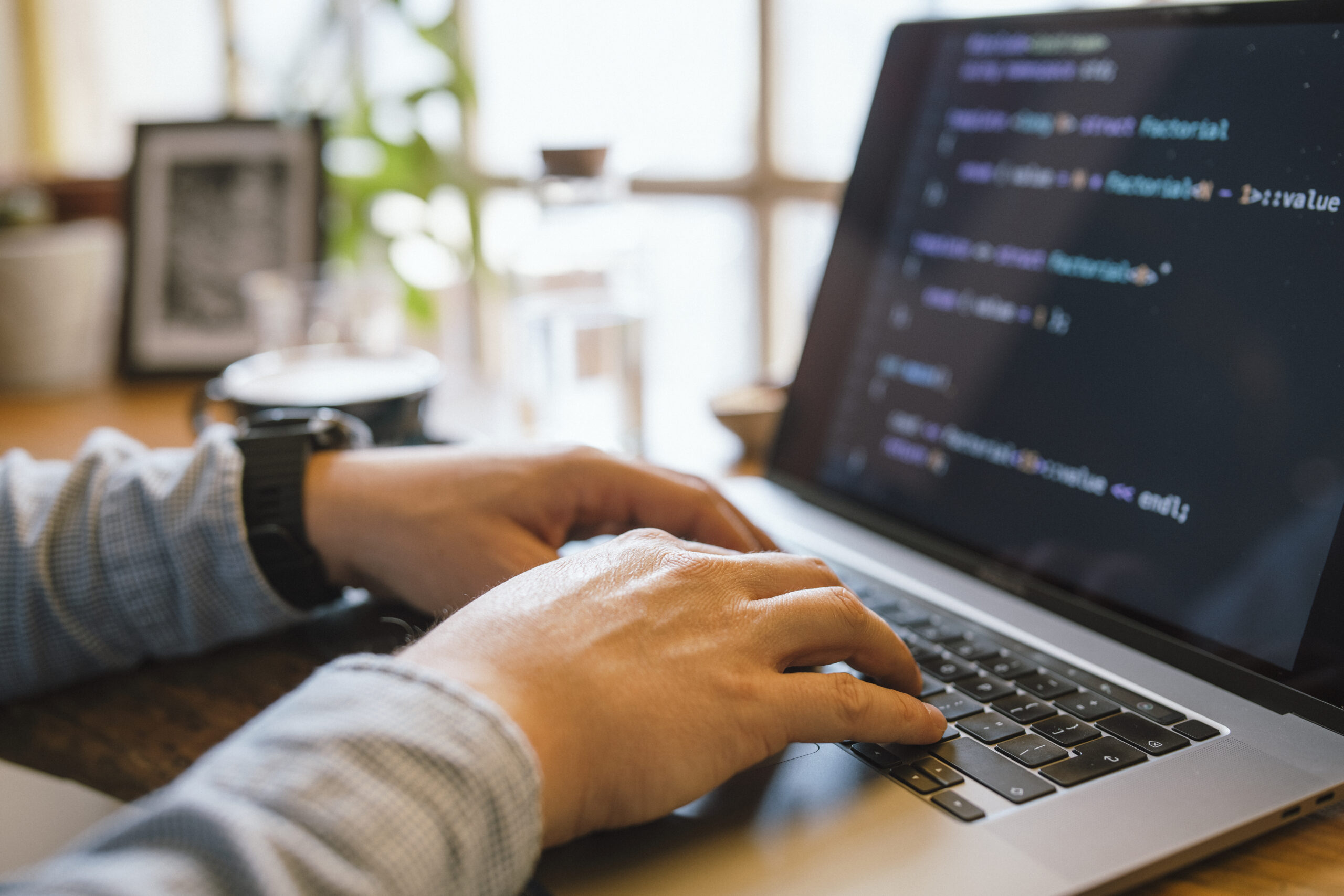
Debugging is one of the most crucial — still often ignored — expertise in the developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Erroneous, and Studying to Feel methodically to resolve difficulties proficiently. Irrespective of whether you are a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and dramatically increase your productiveness. Listed below are a number of methods to help builders level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest ways builders can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though creating code is a single A part of advancement, understanding how to connect with it efficiently throughout execution is Similarly vital. Modern-day advancement environments come Outfitted with effective debugging abilities — but a lot of developers only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications let you set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and in many cases modify code within the fly. When made use of accurately, they let you observe precisely how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch community requests, check out authentic-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can flip annoying UI issues into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate above jogging procedures and memory management. Understanding these instruments can have a steeper Studying curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage devices like Git to understand code background, locate the exact minute bugs were being released, and isolate problematic adjustments.
In the long run, mastering your instruments usually means likely further than default configurations and shortcuts — it’s about developing an intimate understanding of your growth setting making sure that when difficulties occur, you’re not missing in the dark. The better you know your tools, the greater time you could expend resolving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the condition
One of the more critical — and infrequently missed — techniques in powerful debugging is reproducing the challenge. Just before jumping in to the code or creating guesses, builders will need to make a constant environment or state of affairs where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a match of likelihood, often bringing about squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question issues like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater detail you have got, the less complicated it gets to be to isolate the precise circumstances underneath which the bug happens.
When you’ve gathered sufficient facts, attempt to recreate the condition in your local atmosphere. This might mean inputting precisely the same info, simulating identical user interactions, or mimicking technique states. If the issue seems intermittently, consider composing automatic tests that replicate the edge scenarios or state transitions associated. These tests not merely assistance expose the issue and also prevent regressions Later on.
Sometimes, The problem can be environment-certain — it would materialize only on certain working units, browsers, or below certain configurations. Working with tools like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a action — it’s a mentality. It necessitates patience, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. Using a reproducible state of affairs, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and converse more clearly with your group or customers. It turns an abstract complaint right into a concrete challenge — Which’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages in many cases are the most worthy clues a developer has when a thing goes Erroneous. As an alternative to viewing them as aggravating interruptions, developers should discover to treat mistake messages as immediate communications from your method. They often show you just what exactly transpired, wherever it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the information diligently As well as in complete. Many builders, especially when less than time strain, glance at the very first line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the accurate root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the mistake down into pieces. Could it be a syntax mistake, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or functionality induced it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging method.
Some glitches are vague or generic, and in People conditions, it’s essential to look at the context wherein the error transpired. Look at connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These usually precede larger sized issues and provide hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, encouraging you realize what’s taking place under the hood with no need to pause execution or phase with the code line by line.
An excellent logging method begins with understanding what to log and at what stage. Widespread logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data all through progress, Details for standard activities (like effective start-ups), Alert for probable troubles that don’t crack the appliance, ERROR for precise challenges, and Deadly when the system can’t continue on.
Keep away from flooding your logs with abnormal or irrelevant knowledge. Excessive logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, condition adjustments, input/output values, and significant selection details with your code.
Format your log messages Plainly and constantly. Involve context, such as timestamps, ask for IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-imagined-out logging strategy, you could reduce the time it requires to identify challenges, acquire deeper visibility into your apps, and Increase the Over-all maintainability and trustworthiness of one's code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it is a sort of investigation. To correctly discover and deal with bugs, builders must strategy the method just like a detective resolving a secret. This mindset assists stop working advanced issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate information and facts as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s taking place.
Following, kind hypotheses. Question oneself: What could possibly be leading to this behavior? Have any changes recently been built to your codebase? Has this issue happened in advance of below equivalent situations? The goal would be to narrow down possibilities and detect potential culprits.
Then, exam your theories systematically. Seek to recreate the situation in the controlled ecosystem. When you suspect a particular function or ingredient, isolate it and confirm if The problem persists. Like a detective conducting interviews, check with your code issues and Allow the results direct you closer to the reality.
Pay out close awareness to modest specifics. Bugs often conceal in the minimum expected destinations—like a lacking semicolon, an off-by-one mistake, or possibly a race problem. Be complete and individual, resisting the urge to patch the issue with no fully knowledge it. Temporary fixes may possibly hide the true problem, only for it to resurface afterwards.
Finally, retain notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential problems and support Many others comprehend your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering hidden concerns in advanced systems.
Create Exams
Producing checks is among the simplest methods to boost your debugging capabilities and All round progress performance. Checks not only assist catch bugs early but in addition serve as a safety Internet that provides you self confidence when earning changes for your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when an issue occurs.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can rapidly expose whether a selected bit of logic is working as envisioned. Any time a take a look at fails, you promptly know the place to seem, substantially decreasing the time used debugging. Device exams are Specially handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Up coming, integrate integration checks and conclude-to-finish checks into your workflow. These enable make sure several areas of your application get the job done collectively smoothly. They’re specially beneficial for catching bugs that occur in elaborate techniques with multiple parts or expert services interacting. If one thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating assessments also forces you to Assume critically about your code. To check a characteristic properly, you may need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a problem, writing a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails continually, you are able to target correcting the bug and view your examination go when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you capture far more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky concern, it’s effortless to be immersed in the situation—gazing your screen for hours, attempting Remedy right after Alternative. But Probably the most underrated debugging resources is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see the issue from a new perspective.
When you're too near to the code for way too prolonged, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. During this point out, your Mind gets significantly less successful at dilemma-fixing. A short wander, a espresso split, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of a difficulty after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do some thing unrelated to code. It could really feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak here point—it’s a sensible technique. It offers your Mind Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile when you take the time to reflect and evaluate what went Mistaken.
Start by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code opinions, or logging? The responses generally expose blind spots as part of your workflow or being familiar with and assist you Establish much better coding behaviors transferring ahead.
Documenting bugs can be a superb behavior. Maintain a developer journal or preserve a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see patterns—recurring challenges or popular faults—which you could proactively stay away from.
In group environments, sharing what you've figured out from a bug together with your friends is often Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or A fast understanding-sharing session, helping Some others stay away from the same challenge boosts group performance and cultivates a more robust Studying lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from frustration to curiosity. In lieu of dreading bugs, you’ll commence appreciating them as essential areas of your development journey. In spite of everything, many of the greatest builders usually are not those who create great code, but people who consistently discover from their faults.
In the end, Every single bug you fix adds a completely new layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — nevertheless the payoff is big. It makes you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.